UILabel 使用细节
引言
# 一. 自定义间距
# 1. 行间距
# 方法:
/// 改变 label 行间距
- (void)lc_changeLineSpace:(float)space {
NSString *labelText = self.text;
NSMutableAttributedString *attr = [[NSMutableAttributedString alloc] initWithString:labelText];
NSMutableParagraphStyle *paragraphStyle = [[NSMutableParagraphStyle alloc] init];
CGFloat ss = lineSpace - (self.font.lineHeight - self.font.pointSize);
[paragraphStyle setLineSpacing:ss];
[attr addAttribute:NSParagraphStyleAttributeName value:paragraphStyle range:NSMakeRange(0, [labelText length])];
self.attributedText = attr;
[self sizeToFit];
}
# 注意:
如果我们只设置paragraphStyle.lineSpacing = 10
会有问题:
红色区域是默认绘制单行文本占用的区域,可以看到文字的上下是有一些空白的。
红色区域的高度是 label.font.lineHeight
,通过计算就可得到要设置的真实行间距:10 - (label.font.lineHeight - label.font.pointSize);
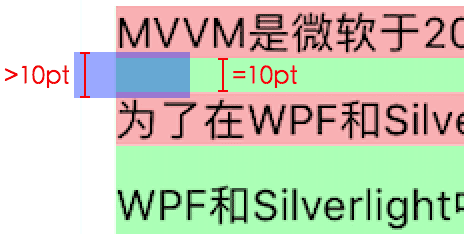
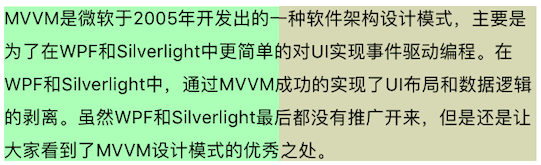
# 2. 字间距
/// 修改 label 字间距
- (void)lc_changeWordSpace:(float)space {
NSString *labelText = self.text;
NSMutableAttributedString *attr = [[NSMutableAttributedString alloc] initWithString:labelText attributes:@{NSKernAttributeName:@(space)}];
NSMutableParagraphStyle *paragraphStyle = [[NSMutableParagraphStyle alloc] init];
[attr addAttribute:NSParagraphStyleAttributeName value:paragraphStyle range:NSMakeRange(0, [labelText length])];
self.attributedText = attr;
[self sizeToFit];
}
# 二. 显示 HTML 数据
使用 富文本 NSHTMLTextDocumentType
实现 :
NSString *htmlStr = @"<h1>Header</h1><h2>Subheader</h2><p>Some <em>text</em></p><img src='http://blogs.babble.com/famecrawler/files/2010/11/mickey_mouse-1097.jpg' width=70 height=100 />";
UILabel *label = [[UILabel alloc] initWithFrame:(CGRectMake(0, 0, 100, 30))];
NSAttributedString *attr = [[NSAttributedString alloc] initWithData:[htmlStr dataUsingEncoding:NSUnicodeStringEncoding]
options:@{NSDocumentTypeDocumentAttribute:NSHTMLTextDocumentType}
documentAttributes:nil
error:nil];
label.attributedText = attr;
# 三. 计算文本高度
注意: 计算高度一定要在给 label 设置了文本之后; 要先确保设置了 width 才能去计算高度;
/**
* 计算 size 要先确保设置了 width
*
* @param lineS 行间距
* @param wordS 字间距
*/
- (CGSize)lc_computeSizeWithLineSpace:(float)lineS
wordSpace:(float)wordS {
// 设置高度宽度的最大限度
CGSize size = CGSizeMake(self.lc_width, MAXFLOAT);
// 计算方式
NSStringDrawingOptions options = NSStringDrawingUsesFontLeading | NSStringDrawingTruncatesLastVisibleLine | NSStringDrawingUsesLineFragmentOrigin;
// 富文本
CGFloat ss = lineS - (self.font.lineHeight - self.font.pointSize);
NSMutableParagraphStyle *paragraphStyle = [[NSMutableParagraphStyle alloc] init];
paragraphStyle.lineSpacing = ss;
NSDictionary *attributes = @{NSFontAttributeName: self.font, // 字号
NSParagraphStyleAttributeName: paragraphStyle, // 行间距
NSKernAttributeName: @(wordS) }; // 字间距
CGRect rect = [self.text boundingRectWithSize:size options:options attributes:attributes context:nil];
return CGSizeMake(self.lc_width, rect.size.height);
}