UIImage 使用细节
引言:
# 一. 清除超出父视图部分
// 清除
self.imgView.clearsContextBeforeDrawing = YES;
# 二. 创建纯色图片
# 1. 创建:
// 创建纯色图片
- (UIImage *)imageWithColor:(UIColor *)color {
CGRect rect = CGRectMake(0, 0, self.view.frame.size.width, self.view.frame.size.height);
UIGraphicsBeginImageContext(rect.size);
CGContextRef context = UIGraphicsGetCurrentContext();
CGContextSetFillColorWithColor(context, [color CGColor]);
CGContextFillRect(context, rect);
UIImage *img = UIGraphicsGetImageFromCurrentImageContext();
UIGraphicsEndImageContext();
return img;
}
# 2. 使用:
UIImageView *imagV =[[UIImageView alloc] initWithFrame:(CGRectMake(200, 400, 100, 100))];
imagV.image = [self imageWithColor:[UIColor blueColor]];
[self.view addSubview:imagV];
# 三. 设置内容模式
self.imgView.contentMode = UIViewContentModeScaleAspectFill;
/// contentMode 主要是用来设置图片的显示方式,如居中、居右,是否缩放等,有以下几个常量可供设定:
UIViewContentModeScaleAspectFit // 图片拉伸至完全显示在UIImageView里面为止(图片不会变形)
UIViewContentModeScaleAspectFill // 图片拉伸至 图片的宽度等于UIImageView的宽度 或者 图片的高度等于UIImageView的高度 为止
UIViewContentModeRedraw // 调用了setNeedsDisplay方法时,就会将图片重新渲染
UIViewContentModeCenter // 居中显示
UIViewContentModeTop // 居上显示
UIViewContentModeBottom // 居下显示
UIViewContentModeLeft // 居左
UIViewContentModeRight // 居右显示
UIViewContentModeTopLeft // 居左上显示
UIViewContentModeTopRight // 居右上显示
UIViewContentModeBottomLeft // 居左下显示
UIViewContentModeBottomRight // 居右下显示
# 1. 注意:
- 以上几个常量,凡是没有带Scale的,当图片尺寸超过 ImageView 尺寸时,只有部分显示在ImageView中。
UIViewContentModeScaleToFill
属性会导致图片变形。UIViewContentModeScaleAspectFit
会保证图片比例不变,而且全部显示在ImageView中,这意味着ImageView会有部分空白。UIViewContentModeScaleAspectFill
也会证图片比例不变,但是是填充整个ImageView的,可能只有部分图片显示出来。
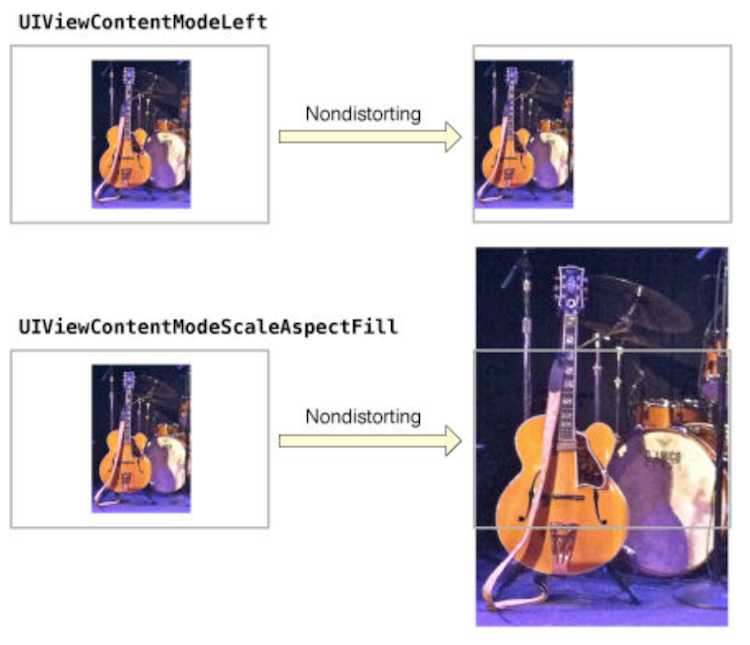
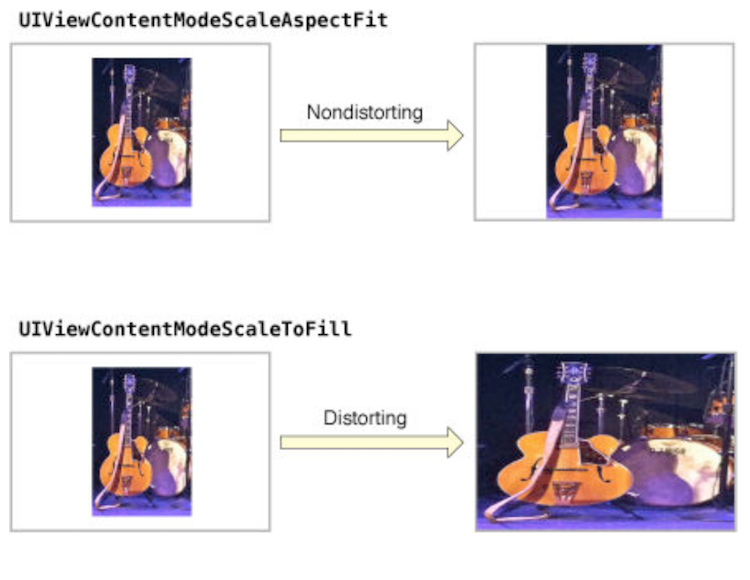
# 四. 图片的加载方式
# 1. 使用 imageName:
加载图片:
- 加载到内存当中之后, 会一直停留在内存中, 不会随着对象销毁而销毁, 加载过的图片, 永远有缓存
- 加载进去图片之后, 占用的内存归系统管理, 我们无法管理
- 相同的图片, 图片不会重复加载
- 加载到内存当中后, 占据内存空间较大
# 2. 使用 imageWithContentsOfFile:
加载图片:
- 加载到内存中后, 占据内存空间较小
- 相同的图片会被重复加载到内存中
- 对象销毁的时候, 加载到内存中的图片会随着一起销毁
# 3. 结论:
- 如果图片较小, 并且使用频繁的图片使用
imageName:
加载(按钮图标/ 主页里面图片), 图片放在Images.xcassets中 - 如果图片较大, 并且使用较少, 使用
imageWithContentsOfFile:
加载(Tom猫/版本新特性/相册), 不要放在Images.xcassets
# 五. 图片存放
我们项目中正常图片会有两种存放方式: 放到 Images.xcassets里面, 直接拖入到项目中
# 1. 放到 Images.xcassets里面:
- 部署版本在 >=iOS8 的时候, 打包的资源包中图片会被放到Assets.car中, 图片被压缩
- 部署版本在 <iOS8 的时候, 打包的资源包中图片会被放在MainBundle中. 图片没有被压缩
# 2. 图片直接拖入到项目中:
- 如果图片直击被拖入到项目中, 无论部署版本是多少, 都会被放到MainBundle里, 图片没有被压缩